Data API Integration
Curveseries Data API
The Curveseries Data API is built to help customers access our data seamlessly to work with internal tools and applications within their organisation.
Note: In order for the API to work, the user needs to be logged in on the Curveseries Desktop.

java api
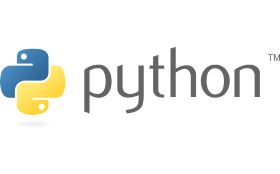
python api
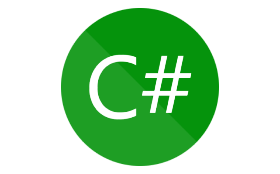
C# Api
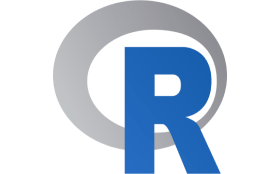
R API
java api
-
1STEP 1:Download this file and unzip to your folders
-
2STEP 2:Import the jar files 'jeromq-0.4.3.jar' and 'csconnect.jar' to your project
-
3STEP 3:Call API in your code in example below
//Start of code
import java.util.Arrays;
import com.curveseries.CSDataAPI;
public class testcs {
public static void main(String[] args) {
//getting historical timeseries
String symbol = "Swap_Nap_FP_2019F.Close";
String start = "01-Jan-2018";
String end = "01-Jul-2018";
String[][] arraylist = CSDataAPI.getCSData(symbol,start,end);
System.out.println(Arrays.deepToString(arraylist));
//getting curve of a particular date
String curve = "Swap_Nap_FP.Close";
String curve_date = "Latest()";
String end_date = "02-Dec-2022";
String[][] arraylistcurve = CSDataAPI.getCSCurve(curve,curve_date,end_date);
System.out.println(Arrays.deepToString(arraylistcurve));
}
}
//End of code
python api
-
1STEP 1:Install pyzmq using command "pip install pyzmq"
-
2STEP 2:Download the corresponding Python version library, unzip and put the pyd in the same folder of your source file
For Python 3.7
For Python 3.8
For Python 3.9
For Python 3.10
For Python 3.11
For Python 3.12 -
3STEP 3:Call API in your code in example below #Start of code import CSDataAPI
#Start of code
import CSDataAPI
equation= "Swap_Nap_FP_2019F.Close"
start_date= "01-Jan-2018"
end_date= "01-Jul-2018"
#getting historical timeseries
result = CSDataAPI.getCSData(equation, start_date, end_date)
print ("Result from getCSData(): ")
for row in result:
print (row)
#getting curve of a particular date
curve= "Swap_Nap_FP.Close"
curve_date= "Latest()"
end_date= "01-Dec-2022"
result = CSDataAPI.getCSCurve(curve, curve_date, end_date)
print ("Result from getCSCurve(): ")
for row in result:
print (row)
//End of code
C# Api
-
1STEP 1: Run the command in NuGet Package Manager in Visual Studio 'Install-Package NetMQ -Version 4.0.1.6'
-
2STEP 2:Download this file and include it in your project reference
-
3STEP 3:Call API in your code in example below
//Start of Code
using CSConnect;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace testcs
{
class testcode
{
static void Main(string[] args)
{String symbol = "Swap_EBOB_FP_2021F.Close";String start = "30-Jan-2020";String end = "30-Nov-2020";String[,] arraylist = CSConnect.CSDataAPI.getCSData(symbol, start, end);int rowLength = arraylist.GetLength(0);int colLength = arraylist.GetLength(1);for (int i = 0; i < rowLength; i++){for (int j = 0; j < colLength; j++)Console.Write(string.Format("{0} ", arraylist[i, j]));Console.Write(Environment.NewLine);}Console.ReadLine();}}}//End of code
R API
-
1STEP 1: Install the rzmq package using 'install.packages("rzmq")'
-
2STEP 2:Download this file and include it in your source reference
-
3STEP 3:Call API in your code in example below
#Start of Code
source("CSDataAPI.R")
#Geting series from Curveseries API
equation= "Swap_Nap_FP_2019F.Close"
start_date= "01-Jan-2018"
end_date= "01-Jul-2018"
arr1 = getcsdata(equation,start_date,end_date)
print(arr1)
#Geting curve from Curveseries API
curve= "Swap_Nap_FP.Close"
curve_date= "Latest()"
end_date= "01-Dec-2022"
arr2 = getcscurve(curve,curve_date,end_date)
print(arr2)
#End of code